第1步:項目
垃圾桶設計為在超聲波距離傳感器檢測到人員時打開。當傳感器沒有檢測到任何人3秒鐘時,它會向后運行電機直到它按下按鈕。溫度和濕度傳感器(DHT)在垃圾桶中記錄條件并計算內容物何時會腐爛。液晶顯示屏顯示時間,直到清空為止。
步驟2:零件清單
1 - Arduino MEGA 2560
1 - 面包板
1 - 10k歐姆電位器
1 - LCD屏幕(與Hitachi HD44780驅動程序兼容)
1 - DHT11
3 - 10k歐姆電阻
1 - 220歐姆電阻
1 - 電機控制L298N帶H橋
1 - 12伏直流電機
1 - 12伏電源
1 - 超聲波傳感器HC-SR04
1 - 按鈕
步驟3:機械設置
為了打開垃圾桶,我們制作了一個機械臂。它有兩個接頭,連接到電機和垃圾桶的蓋子。就像在第一張照片中一樣。
在秒圖中可以看到它安裝在電機上。
對于電機,我們鉆了一些孔并使用了拉鏈。可以看到在第三張照片中安裝在垃圾箱內。手臂粘在蓋子頂部
對于超聲波測距儀,我們在垃圾桶前面鉆了2個孔,直徑= 16mm,間距為1厘米(見4和5圖片)。/p》
對于其他部件,我們剛剛為拉鏈或螺釘鉆孔。在為其鉆孔之后,LCD用螺栓和結安裝在蓋子上。帶拉鏈的arduino和馬達驅動器。
臂部件采用3D打印。原理圖是在Autofusion 360中制作的,并使用了以下文件:
第4步:Fritzing
第一張圖顯示了如何系統已連線。
步驟5:代碼
代碼分為五個不同的功能,每個功能控制一個不同的傳感器或一個傳感器的一部分。為了利用代碼,必須為每個函數創建一個“新選項卡”。
arduino項目文件夾必須包含每個函數的代碼作為文件。
為了程序運行不間斷我們使用millis()和micros()而不是delay()。
該程序還需要以下庫,這些庫都可以在庫管理器中找到:
LiquidCrystal v1.0.7(內置)
DHT傳感器庫v1.3.0
主程序:
//Global variables are defined here. They‘re read through out the entire program.
//Local variables are defined within each function. They can only be changed or read within the function.
//Records whether the ultrasonic sensor has detected something.
//It’s controlled within the DistanceSensor function. It will stay on for four seconds when something is within range.
//Everytime it registers something it refreshes the timer.
boolean Distance_Close;
//Remembers if trashcan is open or closed. One is open and zero is closed.
bool TrashcanState;
//Monitors for local variables.
int ButtonMonitor;
int DistanceMonitor;
int CloseMonitor;
unsigned long TimeOpenMonitor;
unsigned long TimeUsedMonitor;
int DetectedMonitor;
int TemperatureMonitor;
int HumidityMonitor;
//Sets the pin numbers.
const int DCmotorIn1 = 5;
const int DCmotorIn2 = 6;
const int TrigPin = 8;
const int EchoPin = 7;
const int ButtonPin = 9;
const int rs = 13, en = 12, d4 = 11, d5 = 10, d6 = 22, d7 = 24; //Pin numbers for the LCD.
//Libraries, make sure to install all of them.
//All libraries can be found inside the Library Manager.
//“Adafruit Unified Sensor Library” must also be installed in order for dht to work even though it is not loaded.
#include
#include “DHT.h”
#define DHTPIN 3 //Defines which pin should be used for the temperature and humidity sensor
#define DHTTYPE DHT11 //Defines which type of DHT is used. If you have a DHT22 replace DHT11 with DHT22.
DHT dht(DHTPIN, DHTTYPE); //Loads the defined pin and type.
LiquidCrystal lcd(rs, en, d4, d5, d6, d7); //Tells the program which pins are installed.
void setup() {
// Code that needs to be run once:
Serial.begin(9600); //Starts communication with the monitor. Make sure that the monitor have the same BAUD speed.
//Determine if pins are reading or writing.
pinMode(TrigPin,OUTPUT);
pinMode(EchoPin,INPUT);
pinMode(DCmotorIn1, OUTPUT);
pinMode(DCmotorIn2, OUTPUT);
pinMode(ButtonPin,INPUT);
dht.begin();
lcd.begin(16, 2); //Sets the dimension of the LCD
}
void loop() {
// put your main code here, to run repeatedly:
DistanceSensor(); //Constantly checks if someone is near the trashcan
OpenTrashcan(); //Checks if the trashcan should opened.
CloseTrashcan(); //Checks if the trashcan should be closed.
LCD();
TempHumid();
//Creates a table for monitoring all the different variables.
Serial.print(“Trashcan State : ”);
Serial.print(TrashcanState);
Serial.print(“ ”);
Serial.print(“Distance_Close: ” );
Serial.print(Distance_Close);
Serial.print(“ ”);
Serial.print(“Button: ” );
Serial.print(ButtonMonitor);
Serial.print(“ ”);
Serial.print(“Temperature: ” );
Serial.print(TemperatureMonitor);
Serial.print(“ ”);
Serial.print(“Humidity: ” );
Serial.print(HumidityMonitor);
Serial.print(“ ”);
Serial.print(“Distance: ” );
Serial.println(DistanceMonitor);
控制距離傳感器的功能:
int DistanceSensor(){
//Local variables used only in this function
unsigned long TimeUsed;
unsigned long Wait;
unsigned long WaitExtra;
unsigned long TimeOpen;
bool Detected;
long Duration;
int Distance;
TimeUsed = micros(); //Reads how long the arduino have been on in microseconds.
//Writes the local variables into global variables for monitoring
DetectedMonitor = Detected;
DistanceMonitor = Distance;
//Make the ultrasonic sensor send out a sonic wave and starts two timers.
//It is activated after 12 microseconds as the timer is exceeded and the function restarts.
if (WaitExtra 《 TimeUsed){
digitalWrite(TrigPin,HIGH); //Sends out a sonic wave
Wait = TimeUsed + 10; //Takes the current time and adds 10 microseconds to create a timer
WaitExtra = TimeUsed + 12; //Takes the current time and adds 12 to create a timer
}
//Turns off the sonic wave and reads how long it took for it to return.
//Afterwards it calculates the distance it have traveled.
//The distance is calculated in cm.
if (Wait 》= TimeUsed){
digitalWrite(TrigPin,LOW); // Stops the sonic wave
Duration = pulseIn(EchoPin,HIGH); //Reads how long it took for the wave to return in microseconds.
Distance = Duration*0.034/2; //Calculates the distance
}
//Checks if anyone is within a certain distance.
//This can be changed depending on when you want it to trigger.
//Remember this can‘t be less than 2 cm (0.79 inches) unless you use a different sensor.
if ((Distance 》= 10) && (Distance 《= 30)){
Detected = 1; //Something is detected
}
else{
Detected = 0; //Nothing has been detected
}
//Allows the Distance_Close to be set if someone if within the distance set.
//Refreshes if something is detected again.
if (Detected == 1){
TimeOpen = TimeUsed + 4000000; //Refreshes or sets the time that must pass to reset Distance_Close.
}
//Sets Distance_Close to 1, if the time set in detected hasn’t been exceed.
if (TimeOpen 》 TimeUsed){
Distance_Close = 1;
}
//Sets Distance_Close to 0, if the time set in detected has been exceed.
if (TimeOpen 《= TimeUsed){
Distance_Close = 0;
}
}
控制LCD的功能:
int LCD(){
lcd.setCursor(0, 0); //Begins writing at the top row, from the beginning
lcd.print(“Temperature: ”); //Writes “temperature” on the LCD
lcd.print(TemperatureMonitor); //Writes the number measured by the DHT
lcd.setCursor(0,1); //Begins writig at the bottom row, from the beginning
lcd.print(“Humidity: ”); //Writes “humidity” on the LCD
lcd.print(HumidityMonitor); //Writes the number measured by the DHT
}
打開垃圾桶:
bool OpenTrashcan() {
//If someone is detected within a set range in DistanceSensor, the trashcan open.
if ((TrashcanState == 0) && (Distance_Close == 1)){
//Sets the motor to run at full speed in a certain direction. In our case it is CLOCKWISE???
analogWrite(DCmotorIn1, 0);
analogWrite(DCmotorIn2, 255);
//Stops the entire program to make sure it doesn‘t stop while opening.
delay(600);
//After the delay is over the motor is turned off.
analogWrite(DCmotorIn1, 0);
analogWrite(DCmotorIn2, 0);
//Tells the rest of the program that the trashcan is open.
TrashcanState = 1;
}
}
關閉垃圾桶的功能:
bool CloseTrashcan() {
//Local variable to control the button.
int button;
button = digitalRead(9); //Continually check if the button is pressed or not
//Local variable saved as a global varaible for monitoring
ButtonMonitor = button;
//If the trashcan is open and no one has been within the range of the DistanceSensor for the set time the trashcan closes.
if ((TrashcanState == 1) && (Distance_Close == 0)){
//Starts the motor at full power. It turns COUNTER CLOCKWISE???
analogWrite(DCmotorIn1, 255);
analogWrite(DCmotorIn2, 0);
}
//If the trashcan is open and the button is pressed, the trashcan will close.
if ((button == 1) && (TrashcanState == 1)){
//Stops the motor
analogWrite(DCmotorIn1, 0);
analogWrite(DCmotorIn2, 0);
TrashcanState = 0; //Sets the trashcan as open.
}
}
讀取溫度和濕度的函數:
int TempHumid(){
//Local variables used only in this function
unsigned long TimeUsed;
unsigned long Wait;
TimeUsed = millis(); //Counts how long the program have been running in millis
//Reads the temperature and humidity every 2.5 seconds.
if (Wait 《 TimeUsed){
int Humidity;
int Temperature;
Humidity = dht.readHumidity(); //Reads the current humidity in percentage.
Temperature = dht.readTemperature(); //Reads the current temperature in celcius.
HumidityMonitor = Humidity; // The humidity is saved in a global variable for monitoring.
TemperatureMonitor = Temperature; // The temperature is saved in a global variable for monitoring.
Wait = TimeUsed + 2500; //Takes the current time and adds 2500 milliseconds to create a timer.
}
}
為了獲得靈感和幫助,我們使用了以下來源:
超聲波傳感器
https://howtomechatronics.com/tutorials/arduino/u.。.
DHT11
http://www.circuitbasics.com/how-to-set-up-the-dh。 。.
該代碼基于DHT庫提供的示例。它被命名為DHTtester。
L298電機驅動模塊
https://www.instructables.com/id/How-to-use-the-L 。..
LCD
https://www.arduino.cc/en/Tutorial/HelloWorld
第6步:評估
自動垃圾桶功能齊全,但還有很多不足之處。
第一個問題是它無法檢測何時打開。這可以通過安裝伺服而不是直流電機來解決,通過使用可以檢測何時打開的按鈕或類似物。
秒的問題是超聲波傳感器有角度和一些困難時間材料太吸音了。它可以通過使用PIR傳感器來解決。如果安裝了PIR傳感器,它可以成角度以便更有可能檢測到人。
代碼也缺少顯示何時應該清空垃圾桶的部分以及可以判斷垃圾桶何時清空的部分被清空了。
-
智能垃圾桶
+關注
關注
3文章
53瀏覽量
10689
發布評論請先 登錄
相關推薦
垃圾桶滿溢檢測器中溢滿程度監測方案
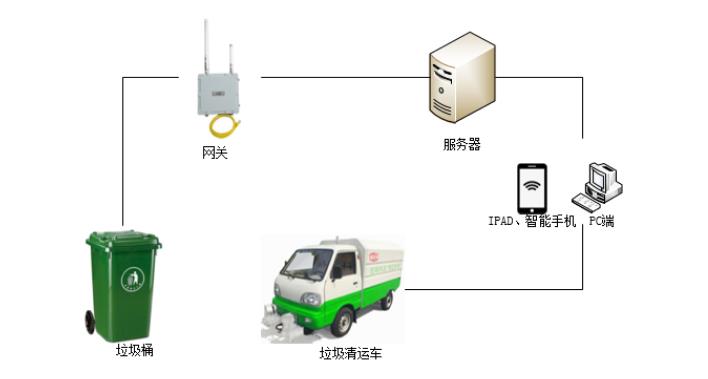
XD08M3232紅外感應單片機在智能垃圾桶抗干擾性分析
XD08M3232接近感應單片機在智能垃圾桶抗干擾性分析
化糞池滿溢檢測器(NB-IoT)IDM-ET34T
FlexLua低代碼零基礎開發智能垃圾桶產品原型(接入機智云)
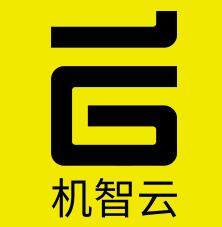
基于PYNQ的智能垃圾分類系統
RFID智慧環衛護航城市垃圾精細化管理
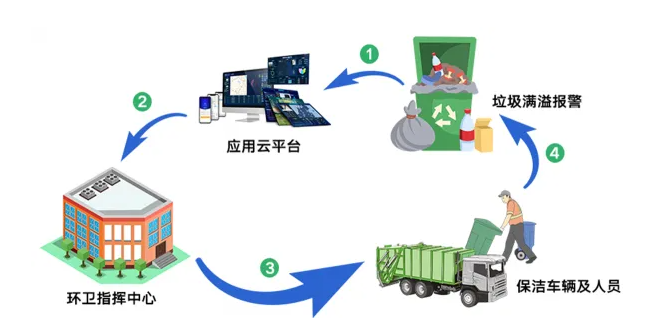
基于機智云物聯網平臺的智能垃圾回收箱與控制系統研究
智能垃圾桶,讓生活更便捷——紅外測距模塊WTU201F2B004引領革新
AI垃圾溢出識別攝像機
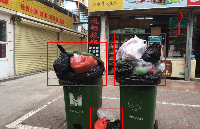
評論