電路圖和說明
4X4鍵盤有8個(gè)引腳需要連接到從D2到D9的Arduino引腳,如下所示:
然后,將LCD連接到Arduino,如下所示:
除了數(shù)字按鈕之外的按鈕將執(zhí)行以下任務(wù):
‘A’用于添加
‘B’用于減法
‘C’用于清除
‘D’用于劃分
‘*’用于乘法
完整的電路圖如下所示。
Arduino計(jì)算器圖。
代碼細(xì)分和演練
我們來看看查看該項(xiàng)目所需的代碼以及每個(gè)代碼段的作用。
首先,您需要為鍵盤和I2C LCD顯示添加庫(kù)。使用的LCD顯示器通過I2C通信與UNO配合使用,因此使用允許在Arduino上進(jìn)行I2C通信的線程庫(kù)。
然后,按照4X4鍵盤的引腳連接和鍵盤的說明進(jìn)行操作按鈕執(zhí)行操作。
#include
#include
#include
const byte ROWS = 4;
const byte COLS = 4;
char keys[ROWS][COLS] = {
{‘1’, ‘2’, ‘3’, ‘+’},
{‘4’, ‘5’, ‘6’, ‘-’},
{‘7’, ‘8’, ‘9’, ‘C’},
{‘*’, ‘0’, ‘=’, ‘/’}
};
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2};
在設(shè)置功能中,顯示屏將顯示“MakerPro的Arduino計(jì)算器”。
lcd.begin();
lcd.print(“Arduino Calculator”);
lcd.setCursor(0, 1);
lcd.print(“by MakerPro”);
delay(1000);
scrollDisplay();
clr();
在循環(huán)功能中,我們先來得到按下的鍵然后我們需要檢查按下的鍵是否是數(shù)字鍵。如果是數(shù)字,則它將存儲(chǔ)在firstNum字符串中。
char newKey = myKeypad.getKey();
if (newKey != NO_KEY && (newKey == ‘1’ || newKey == ‘2’ || newKey == ‘3’ || newKey == ‘4’ || newKey == ‘5’ || newKey == ‘6’ || newKey == ‘7’ || newKey == ‘8’ || newKey == ‘9’ || newKey == ‘0’)) {
if (firstNumState == true) {
firstNum = firstNum + newKey;
lcd.print(newKey);
}
else {
secondNum = secondNum + newKey;
lcd.print(newKey);
}
如果按下的鍵不是數(shù)字,請(qǐng)檢查是否為‘+’,‘ - ’,‘/’,‘*’(在thekeypad上,這些鍵是‘A’,‘B’,‘D’,‘*’)。如果它來自這些鍵,我們將存儲(chǔ)稍后將使用的值。它還會(huì)將firstNum設(shè)置為false,這意味著我們現(xiàn)在將得到第二個(gè)數(shù)字。
現(xiàn)在,其他數(shù)值將存儲(chǔ)在secondNum字符串中。
if (newKey != NO_KEY && (newKey == ‘+’ || newKey == ‘-’ || newKey == ‘*’ || newKey == ‘/’)) {
if (firstNumState == true) {
operatr = newKey;
firstNumState = false;
lcd.setCursor(15, 0);
lcd.print(operatr);
lcd.setCursor(5, 1);
}
}
最后,我們?cè)O(shè)置它,所以如果按下的鍵不是來自操作鍵,它將檢查它是否是‘=’。如果是這個(gè)鍵,那么它將對(duì)第一個(gè)和第二個(gè)數(shù)字執(zhí)行存儲(chǔ)操作并輸出結(jié)果。
設(shè)置完代碼后,計(jì)算器將能夠執(zhí)行方程式。
if (newKey != NO_KEY && newKey == ‘=’) {
if (operatr == ‘+’) {
result = firstNum.toFloat() + secondNum.toFloat();
}
if (operatr == ‘-’) {
result = firstNum.toFloat() - secondNum.toFloat();
}
if (operatr == ‘*’) {
result = firstNum.toFloat() * secondNum.toFloat();
}
if (operatr == ‘/’) {
result = firstNum.toFloat() / secondNum.toFloat();
}
lcd.clear();
lcd.setCursor(0, 0);
lcd.print(firstNum);
lcd.print(operatr);
lcd.print(secondNum);
lcd.setCursor(0, 1);
lcd.print(“=”);
lcd.print(result);
firstNumState = true;
}
And if the key will be ‘C’, then it will clear the display screen.
if (newKey != NO_KEY && newKey == ‘C’) {
clr();
}
完整計(jì)算器項(xiàng)目代碼
#include
#include
#include
const byte ROWS = 4;
const byte COLS = 4;
char keys[ROWS][COLS] = {
{‘1’, ‘2’, ‘3’, ‘+’},
{‘4’, ‘5’, ‘6’, ‘-’},
{‘7’, ‘8’, ‘9’, ‘C’},
{‘*’, ‘0’, ‘=’, ‘/’}
};
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2};
// Created instances
Keypad myKeypad = Keypad(makeKeymap(keys), rowPins, colPins, ROWS, COLS);
LiquidCrystal_I2C lcd(0x27, 16, 2);
boolean firstNumState = true;
String firstNum = “”;
String secondNum = “”;
float result = 0.0;
char operatr = ‘ ’;
void setup() {
lcd.begin();
lcd.setCursor(0, 0);
lcd.print(“Arduino Calculator”);
lcd.setCursor(0, 1);
lcd.print(“by MakerPro”);
delay(1000);
scrollDisplay();
clr();
}
void loop() {
char newKey = myKeypad.getKey();
if (newKey != NO_KEY && (newKey == ‘1’ || newKey == ‘2’ || newKey == ‘3’ || newKey == ‘4’ || newKey == ‘5’ || newKey == ‘6’ || newKey == ‘7’ || newKey == ‘8’ || newKey == ‘9’ || newKey == ‘0’)) {
if (firstNumState == true) {
firstNum = firstNum + newKey;
lcd.print(newKey);
}
else {
secondNum = secondNum + newKey;
lcd.print(newKey);
}
}
if (newKey != NO_KEY && (newKey == ‘+’ || newKey == ‘-’ || newKey == ‘*’ || newKey == ‘/’)) {
if (firstNumState == true) {
operatr = newKey;
firstNumState = false;
lcd.setCursor(15, 0);
lcd.print(operatr);
lcd.setCursor(5, 1);
}
}
if (newKey != NO_KEY && newKey == ‘=’) {
if (operatr == ‘+’) {
result = firstNum.toFloat() + secondNum.toFloat();
}
if (operatr == ‘-’) {
result = firstNum.toFloat() - secondNum.toFloat();
}
if (operatr == ‘*’) {
result = firstNum.toFloat() * secondNum.toFloat();
}
if (operatr == ‘/’) {
result = firstNum.toFloat() / secondNum.toFloat();
}
lcd.clear();
lcd.setCursor(0, 0);
lcd.print(firstNum);
lcd.print(operatr);
lcd.print(secondNum);
lcd.setCursor(0, 1);
lcd.print(“=”);
lcd.print(result);
firstNumState = true;
}
if (newKey != NO_KEY && newKey == ‘C’) {
clr();
}
}
void scrollDisplay() {
// scroll 13 positions (string length) to the left
// to move it offscreen left:
for (int positionCounter = 0; positionCounter 《 3; positionCounter++) {
// scroll one position left:
lcd.scrollDisplayLeft();
// wait a bit:
delay(300);
}
delay(1000);
// scroll 29 positions (string length + display length) to the right
// to move it offscreen right:
for (int positionCounter = 0; positionCounter 《 3; positionCounter++) {
// scroll one position right:
lcd.scrollDisplayRight();
// wait a bit:
delay(300);
}
delay(2000);
}
void clr() {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print(“1st: ”);
lcd.setCursor(12, 0);
lcd.print(“op ”);
lcd.setCursor(0, 1);
lcd.print(“2nd: ”);
lcd.setCursor(5, 0);
firstNum = “”;
secondNum = “”;
result = 0;
operatr = ‘ ’;
}
-
計(jì)算器
+關(guān)注
關(guān)注
16文章
438瀏覽量
37499 -
Arduino
+關(guān)注
關(guān)注
188文章
6477瀏覽量
188050
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
LP光纖模式計(jì)算器
熱敏電阻系數(shù)計(jì)算器工具指南-BQ769x2
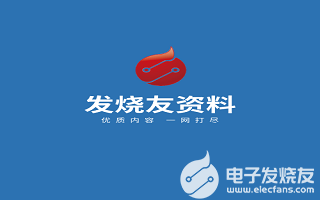
基于FPGA的計(jì)算器設(shè)計(jì)
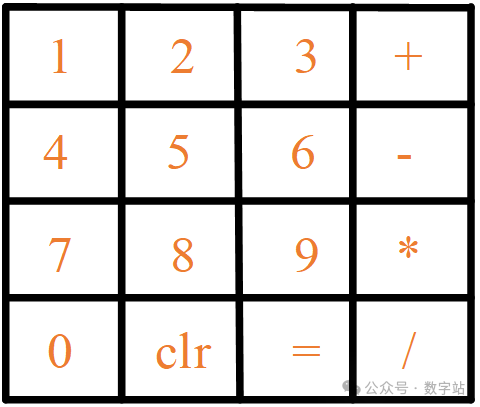
開源項(xiàng)目!基于 Arduino DIY 漂亮的宏機(jī)械鍵盤
OC5865X資料(參數(shù)計(jì)算器&原理圖)
SN65LVCP404千兆位4x4交叉點(diǎn)開關(guān)數(shù)據(jù)表
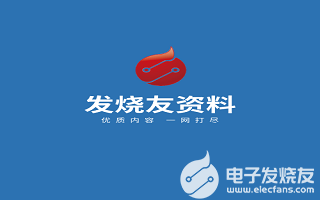
DS25CP104A/CP114 3.125 Gbps 4x4 LVDS交叉點(diǎn)開關(guān)數(shù)據(jù)表
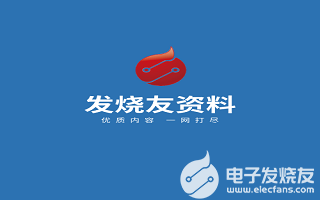
DS10CP154A 1.5Gbps 4x4 LVDS交叉點(diǎn)開關(guān)數(shù)據(jù)表
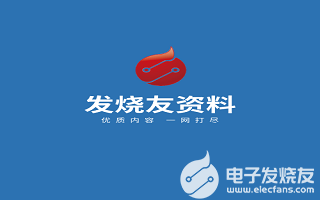
LVDS 4x4交叉點(diǎn)開關(guān)SN65LVDS250數(shù)據(jù)表
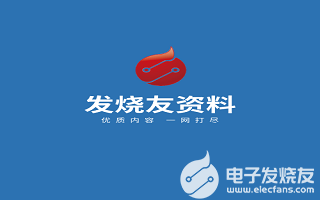
RUCKUS R760資料:室內(nèi) Wi-Fi 6E 4x4:4 接入點(diǎn),8.35 Gbps數(shù)據(jù)速率
stm32f100怎樣用重映射功能?
OpenHarmony開發(fā)案例:【分布式計(jì)算器】
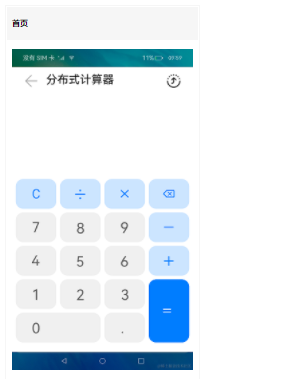
AWTK 開源串口屏開發(fā)(13) - 計(jì)算器應(yīng)用
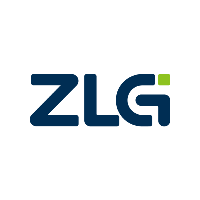
評(píng)論