一、導入elasticsearch依賴
在pom.xml里加入如下依賴
org.springframework.boot spring-boot-starter-data-elasticsearch
非常重要:檢查依賴版本是否與你當前所用的版本是否一致,如果不一致,會連接失敗!
基于 Spring Boot + MyBatis Plus + Vue & Element 實現的后臺管理系統 + 用戶小程序,支持 RBAC 動態權限、多租戶、數據權限、工作流、三方登錄、支付、短信、商城等功能
項目地址:https://github.com/YunaiV/ruoyi-vue-pro
視頻教程:https://doc.iocoder.cn/video/
二、創建高級客戶端
importorg.apache.http.HttpHost; importorg.elasticsearch.client.RestClient; importorg.elasticsearch.client.RestHighLevelClient; importorg.springframework.context.annotation.Bean; importorg.springframework.context.annotation.Configuration; @Configuration publicclassElasticSearchClientConfig{ @Bean publicRestHighLevelClientrestHighLevelClient(){ RestHighLevelClientclient=newRestHighLevelClient( RestClient.builder( newHttpHost("服務器IP",9200,"http"))); returnclient; } }
基于 Spring Cloud Alibaba + Gateway + Nacos + RocketMQ + Vue & Element 實現的后臺管理系統 + 用戶小程序,支持 RBAC 動態權限、多租戶、數據權限、工作流、三方登錄、支付、短信、商城等功能
項目地址:https://github.com/YunaiV/yudao-cloud
視頻教程:https://doc.iocoder.cn/video/
三、基本用法
1.創建、判斷存在、刪除索引
importorg.elasticsearch.action.admin.indices.delete.DeleteIndexRequest; importorg.elasticsearch.action.support.master.AcknowledgedResponse; importorg.elasticsearch.client.RequestOptions; importorg.elasticsearch.client.RestHighLevelClient; importorg.elasticsearch.client.indices.CreateIndexRequest; importorg.elasticsearch.client.indices.CreateIndexResponse; importorg.elasticsearch.client.indices.GetIndexRequest; importorg.junit.jupiter.api.Test; importorg.springframework.beans.factory.annotation.Autowired; importorg.springframework.boot.test.context.SpringBootTest; importjava.io.IOException; @SpringBootTest classElasticsearchApplicationTests{ @Autowired privateRestHighLevelClientrestHighLevelClient; @Test voidtestCreateIndex()throwsIOException{ //1.創建索引請求 CreateIndexRequestrequest=newCreateIndexRequest("ljx666"); //2.客戶端執行請求IndicesClient,執行create方法創建索引,請求后獲得響應 CreateIndexResponseresponse= restHighLevelClient.indices().create(request,RequestOptions.DEFAULT); System.out.println(response); } @Test voidtestExistIndex()throwsIOException{ //1.查詢索引請求 GetIndexRequestrequest=newGetIndexRequest("ljx666"); //2.執行exists方法判斷是否存在 booleanexists=restHighLevelClient.indices().exists(request,RequestOptions.DEFAULT); System.out.println(exists); } @Test voidtestDeleteIndex()throwsIOException{ //1.刪除索引請求 DeleteIndexRequestrequest=newDeleteIndexRequest("ljx666"); //執行delete方法刪除指定索引 AcknowledgedResponsedelete=restHighLevelClient.indices().delete(request,RequestOptions.DEFAULT); System.out.println(delete.isAcknowledged()); } }
2.對文檔的CRUD
創建文檔:
注意:如果添加時不指定文檔ID,他就會隨機生成一個ID,ID唯一。
創建文檔時若該ID已存在,發送創建文檔請求后會更新文檔中的數據。
@Test voidtestAddUser()throwsIOException{ //1.創建對象 Useruser=newUser("Go",21,newString[]{"內卷","吃飯"}); //2.創建請求 IndexRequestrequest=newIndexRequest("ljx666"); //3.設置規則PUT/ljx666/_doc/1 //設置文檔id=6,設置超時=1s等,不設置會使用默認的 //同時支持鏈式編程如request.id("6").timeout("1s"); request.id("6"); request.timeout("1s"); //4.將數據放入請求,要將對象轉化為json格式 //XContentType.JSON,告訴它傳的數據是JSON類型 request.source(JSONValue.toJSONString(user),XContentType.JSON); //5.客戶端發送請求,獲取響應結果 IndexResponseindexResponse=restHighLevelClient.index(request,RequestOptions.DEFAULT); System.out.println(indexResponse.toString()); System.out.println(indexResponse.status()); }
獲取文檔中的數據:
@Test voidtestGetUser()throwsIOException{ //1.創建請求,指定索引、文檔id GetRequestrequest=newGetRequest("ljx666","1"); GetResponsegetResponse=restHighLevelClient.get(request,RequestOptions.DEFAULT); System.out.println(getResponse);//獲取響應結果 //getResponse.getSource()返回的是Map集合 System.out.println(getResponse.getSourceAsString());//獲取響應結果source中內容,轉化為字符串 }
更新文檔數據:
注意:需要將User對象中的屬性全部指定值,不然會被設置為空,如User只設置了名稱,那么只有名稱會被修改成功,其他會被修改為null。
@Test voidtestUpdateUser()throwsIOException{ //1.創建請求,指定索引、文檔id UpdateRequestrequest=newUpdateRequest("ljx666","6"); Useruser=newUser("GoGo",21,newString[]{"內卷","吃飯"}); //將創建的對象放入文檔中 request.doc(JSONValue.toJSONString(user),XContentType.JSON); UpdateResponseupdateResponse=restHighLevelClient.update(request,RequestOptions.DEFAULT); System.out.println(updateResponse.status());//更新成功返回OK }
刪除文檔:
@Test voidtestDeleteUser()throwsIOException{ //創建刪除請求,指定要刪除的索引與文檔ID DeleteRequestrequest=newDeleteRequest("ljx666","6"); DeleteResponseupdateResponse=restHighLevelClient.delete(request,RequestOptions.DEFAULT); System.out.println(updateResponse.status());//刪除成功返回OK,沒有找到返回NOT_FOUND }
3.批量CRUD數據
這里只列出了批量插入數據,其他與此類似
注意:hasFailures()方法是返回是否失敗,即它的值為false時說明上傳成功
@Test voidtestBulkAddUser()throwsIOException{ BulkRequestbulkRequest=newBulkRequest(); //設置超時 bulkRequest.timeout("10s"); ArrayListlist=newArrayList<>(); list.add(newUser("Java",25,newString[]{"內卷"})); list.add(newUser("Go",18,newString[]{"內卷"})); list.add(newUser("C",30,newString[]{"內卷"})); list.add(newUser("C++",26,newString[]{"內卷"})); list.add(newUser("Python",20,newString[]{"內卷"})); intid=1; //批量處理請求 for(Useru:list){ //不設置id會生成隨機id bulkRequest.add(newIndexRequest("ljx666") .id(""+(id++)) .source(JSONValue.toJSONString(u),XContentType.JSON)); } BulkResponsebulkResponse=restHighLevelClient.bulk(bulkRequest,RequestOptions.DEFAULT); System.out.println(bulkResponse.hasFailures());//是否執行失敗,false為執行成功 }
4.查詢所有、模糊查詢、分頁查詢、排序、高亮顯示
@Test voidtestSearch()throwsIOException{ SearchRequestsearchRequest=newSearchRequest("ljx666");//里面可以放多個索引 SearchSourceBuildersourceBuilder=newSearchSourceBuilder();//構造搜索條件 //此處可以使用QueryBuilders工具類中的方法 //1.查詢所有 sourceBuilder.query(QueryBuilders.matchAllQuery()); //2.查詢name中含有Java的 sourceBuilder.query(QueryBuilders.multiMatchQuery("java","name")); //3.分頁查詢 sourceBuilder.from(0).size(5); //4.按照score正序排列 //sourceBuilder.sort(SortBuilders.scoreSort().order(SortOrder.ASC)); //5.按照id倒序排列(score會失效返回NaN) //sourceBuilder.sort(SortBuilders.fieldSort("_id").order(SortOrder.DESC)); //6.給指定字段加上指定高亮樣式 HighlightBuilderhighlightBuilder=newHighlightBuilder(); highlightBuilder.field("name").preTags("").postTags(""); sourceBuilder.highlighter(highlightBuilder); searchRequest.source(sourceBuilder); SearchResponsesearchResponse=restHighLevelClient.search(searchRequest,RequestOptions.DEFAULT); //獲取總條數 System.out.println(searchResponse.getHits().getTotalHits().value); //輸出結果數據(如果不設置返回條數,大于10條默認只返回10條) SearchHit[]hits=searchResponse.getHits().getHits(); for(SearchHithit:hits){ System.out.println("分數:"+hit.getScore()); Mapsource=hit.getSourceAsMap(); System.out.println("index->"+hit.getIndex()); System.out.println("id->"+hit.getId()); for(Map.Entry s:source.entrySet()){ System.out.println(s.getKey()+"--"+s.getValue()); } } }
四、總結
1.大致流程
創建對應的請求 --> 設置請求(添加規則,添加數據等) --> 執行對應的方法(傳入請求,默認請求選項)–> 接收響應結果(執行方法返回值)–> 輸出響應結果中需要的數據(source,status等)
2.注意事項
如果不指定id,會自動生成一個隨機id
正常情況下,不應該這樣使用new IndexRequest(“ljx777”),如果索引發生改變了,那么代碼都需要修改,可以定義一個枚舉類或者一個專門存放常量的類,將變量用final static等進行修飾,并指定索引值。其他地方引用該常量即可,需要修改也只需修改該類即可。
elasticsearch相關的東西,版本都必須一致,不然會報錯
elasticsearch很消耗內存,建議在內存較大的服務器上運行elasticsearch,否則會因為內存不足導致elasticsearch自動killed。
審核編輯:劉清
-
RBAC
+關注
關注
0文章
44瀏覽量
10185 -
SpringBoot
+關注
關注
0文章
175瀏覽量
374
原文標題:SpringBoot+ElasticSearch 實現模糊查詢,批量CRUD,排序,分頁,高亮
文章出處:【微信號:芋道源碼,微信公眾號:芋道源碼】歡迎添加關注!文章轉載請注明出處。
發布評論請先 登錄
SpringBoot整合ElasticSearch
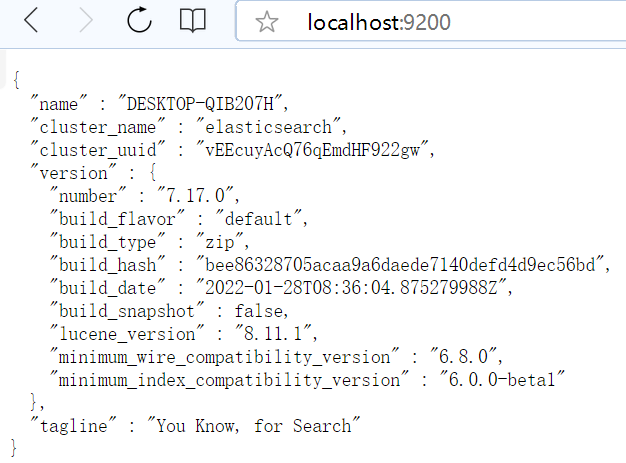
分析一下MySQL數據庫與ElasticSearch的實際應用
為什么ElasticSearch復雜條件查詢比MySQL好?
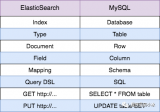
ElasticSearch是什么?應用場景是什么?
SpringBoot模板分類樹查詢功能介紹
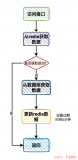
SpringBoot 連接ElasticSearch的使用方式
Redis的分頁+多條件模糊查詢組合實現方案
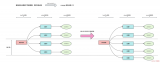
評論